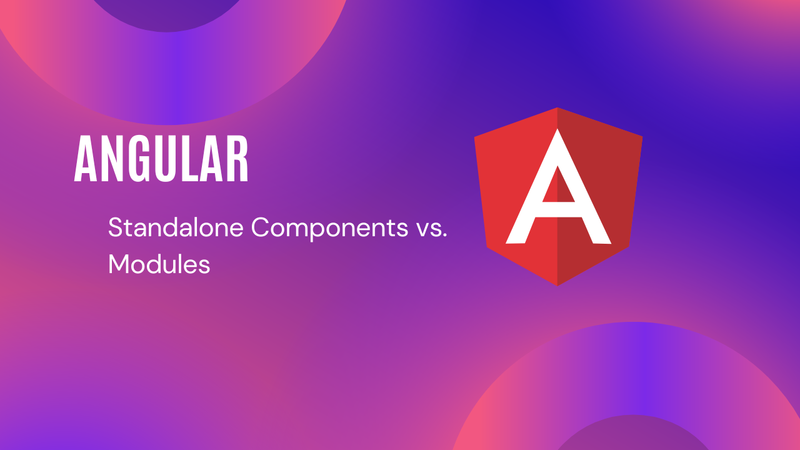
Standalone Components vs. Modules in Angular 17
Introduction
With Angular 17, standalone components became the default when creating a new project using the CLI. You can still create projects using the modular approach, or even combine both. This shift has rendered many online guides seemingly obsolete, but they remain useful with some adjustments.
Objective of the Article
This article aims to clarify the differences between standalone components and modules in Angular 17. We will also explore practical examples and solutions to common errors.
Angular 17 Changes
1. What Has Been Before?
Traditionally, Angular relied on NgModules to group components, directives, services, and other elements, creating a modular structure for applications. This approach helped manage dependencies and organize code. Components, directives, and pipes had to be declared in a module to be used. Dependency injection was primarily handled at the module level.
2. What Happened?
With Angular 17, standalone components were introduced as a standard feature, although they have been present since Angular 14. This shift aimed to simplify development by reducing boilerplate code and making dependency management more straightforward. Standalone components can be used without the need for NgModules, providing a more flexible and streamlined development process. Both approaches are still valid.
Understanding the differences
1. Understanding Modules
Modules in Angular are used to organize and encapsulate related functionality. They serve several purposes:
- Grouping related components, directives, and services
- Managing dependencies between different parts of an application
- Providing a mechanism for lazy-loading features
A typical Angular module looks like this:
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { MyComponent } from './my.component';
import { MyService } from './my.service';
@NgModule({
imports: [CommonModule],
declarations: [MyComponent],
providers: [MyService],
exports: [MyComponent]
})
export class MyModule { }
Modules define a compilation context for their components, allowing Angular to understand how components relate to each other and how to resolve dependencies.
2. Understanding Standalone Components
Standalone components are a new way to define Angular components without the need for an NgModule. They are self-contained and can be used directly in other parts of your application. Here’s an example:
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
@Component({
selector: 'app-my-standalone',
standalone: true,
imports: [CommonModule],
template: '<h1>Hello, I'm a standalone component!</h1>'
})
export class MyStandaloneComponent { }
The key differences are:
- The
standalone: true
property in the@Component
decorator - Direct import of other modules or components in the
imports
array - No need for a separate NgModule declaration
3. Pros vs Cons
Modules:
- Pros:
- Organized structure for large applications
- Clear separation of concerns
- Easier lazy-loading of feature modules
- Cons:
- Can lead to “module hell” in complex applications
- Overhead for smaller applications
- More boilerplate code
Standalone Components:
- Pros:
- Simplified application structure
- Easier to understand and maintain
- More flexible composition of components
- Cons:
- Potential for less organized code in large applications
- Changes to how lazy-loading is implemented
- Learning curve for developers used to module-based architecture
Implementations
1. Implementing modules
To implement a module-based approach:
- Create a new module file (e.g.,
feature.module.ts
) - Define your NgModule with necessary imports, declarations, and exports
- Create components, services, etc., and include them in the module
- Import the module where needed (usually in
app.module.ts
or another feature module)
Example:
// feature.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { FeatureComponent } from './feature.component';
@NgModule({
imports: [CommonModule],
declarations: [FeatureComponent],
exports: [FeatureComponent]
})
export class FeatureModule { }
// app.module.ts
import { NgModule } from '@angular/core';
import { FeatureModule } from './feature/feature.module';
@NgModule({
imports: [FeatureModule],
// ...
})
export class AppModule { }
2. Implementing Standalone Components
To implement standalone components:
- Create your component file
- Add
standalone: true
to the@Component
decorator - Import necessary dependencies directly in the component
- Use the component directly in other parts of your application
Example:
// standalone.component.ts
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
@Component({
selector: 'app-standalone',
standalone: true,
imports: [CommonModule],
template: '<p>I'm a standalone component</p>'
})
export class StandaloneComponent { }
// app.component.ts
import { Component } from '@angular/core';
import { StandaloneComponent } from './standalone.component';
@Component({
selector: 'app-root',
standalone: true,
imports: [StandaloneComponent],
template: '<app-standalone></app-standalone>'
})
export class AppComponent { }
3. The Hybrid Approach
You can combine both approaches in a single application:
- Use standalone components for simpler, self-contained features
- Use modules for larger, more complex features that benefit from encapsulation
- Import standalone components into modules when necessary
Example:
// feature.module.ts
import { NgModule } from '@angular/core';
import { StandaloneComponent } from './standalone.component';
import { ModularComponent } from './modular.component';
@NgModule({
imports: [StandaloneComponent],
declarations: [ModularComponent],
exports: [StandaloneComponent, ModularComponent]
})
export class FeatureModule { }
Dependency injection in standalone applications
In standalone applications, dependency injection is handled differently:
- Services can be provided directly in standalone components:
@Component({
// ...
providers: [MyService]
})
export class StandaloneComponent { }
- For application-wide services, use
providedIn: 'root'
:
@Injectable({
providedIn: 'root'
})
export class GlobalService { }
- For lazy-loaded routes, provide services in the route configuration:
const routes: Routes = [{
path: 'lazy',
loadComponent: () => import('./lazy.component').then(m => m.LazyComponent),
providers: [LazyService]
}];
Common errors and solutions.
- Error: Can’t bind to ‘X’ since it isn’t a known property of ‘Y’
- Solution: Ensure you’ve imported the necessary modules or standalone components that provide the directive or component you’re trying to use.
- Error: No provider for X
- Solution: Check that you’ve properly provided the service, either in the component, a parent component, or at the root level.
- Error: X is not a known element
- Solution: Make sure you’ve imported the component or added it to the
imports
array if it’s a standalone component.
- Solution: Make sure you’ve imported the component or added it to the
For more detailed information, you can refer to the Angular University guide on standalone components and the official Angular documentation.
Ready to get started?
I'd love to hear about your project. Let's chat!